Follow along in this video
This post shows you how we create custom error pages in Umbraco 10 and above at ClerksWell
1. Update the Configure method in the Startup.cs file to send error traffic to the URL /error
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/error");
}
Update the configure method in the Startup.cs file
2. Create a controller to handle the traffic sent to the error page.
using Microsoft.AspNetCore.Mvc;
namespace MyProject.Controllers;
public class ErrorController : Controller
{
[Route("error")]
public IActionResult Index()
{
switch (Response.StatusCode)
{
case StatusCodes.Status200OK:
case StatusCodes.Status500InternalServerError:
return Redirect("/500");
default:
return Redirect("/404");
}
}
}
ErrorController to handle traffic sent to /error
3. Create a Content Type in Umbraco calledĀ Error PageĀ with 2 properties:
Error Status Code a dropdown with the status codes you would like to support
Error Message a message you would like to display on the error page
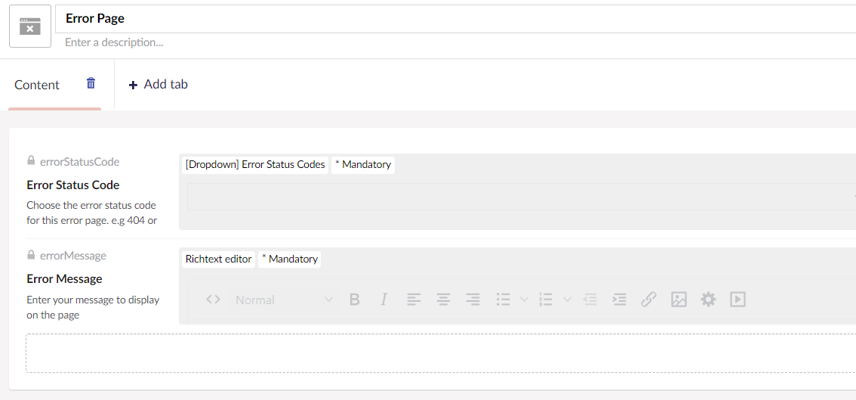
Error Page content type

Error Status Code property
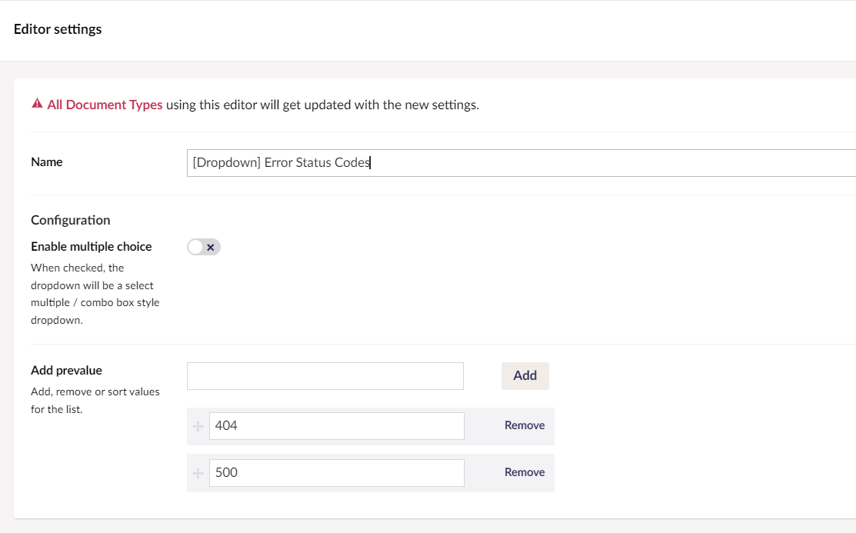
Status Code Dropdown data type
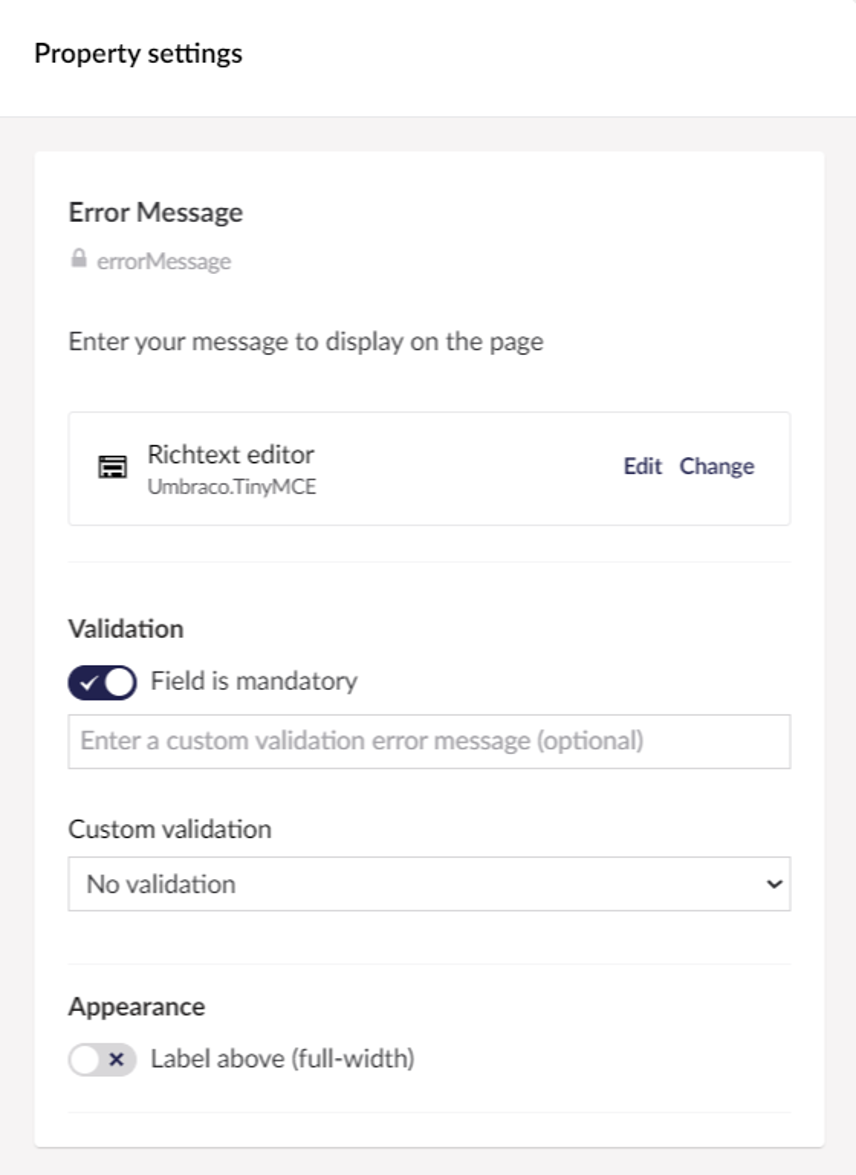
Error message property
4. Allow the new Error Page content type to be a child of your home page content type
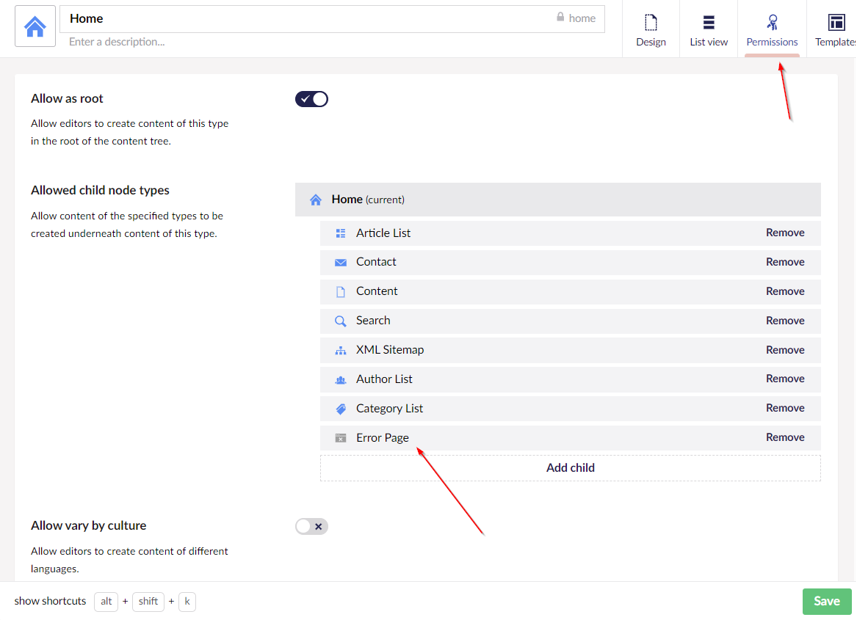
Set permissions
5. Create a page for each of the error status codes you want to support
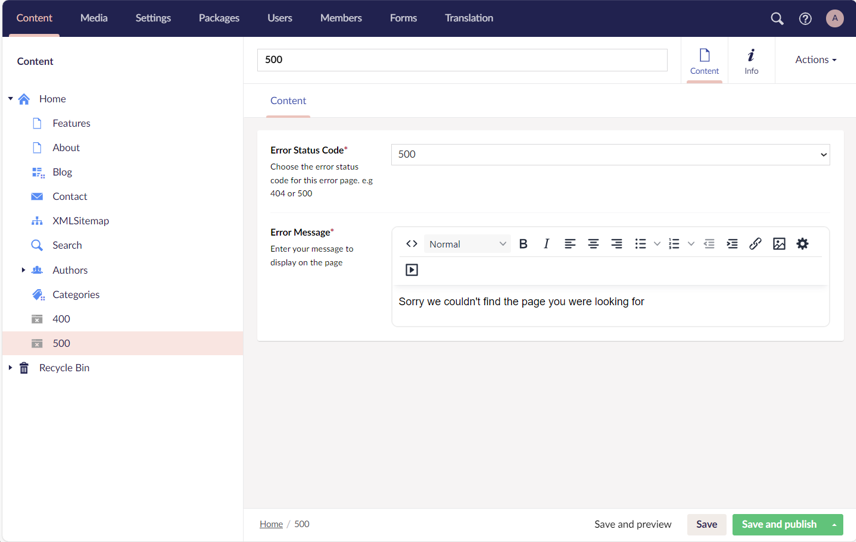
Create the content items for the error pages
6. The last step is to create a render controller for the error page so we can intercept the traffic and apply the correct status code when the page renders.
Before you do this you will need to make sure you are using ModelsBuilder, ideally SourceCodeAuto or SourceCodeManual mode. You will need to generate your ModelsBuilder models first or ErrorPage model won't be recognised in the code.
If you need help getting ModelsBuilder setup, here are the appsettings I am using for models builder
"Umbraco": {
"CMS": {
"ModelsBuilder": {
"ModelsMode": "SourceCodeManual",
"ModelsDirectory": "~/Models/Generated"
}
}
}
Here are the appsettings I am using for models builder
namespace MyProject.Controllers;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.ViewEngines;
using Umbraco.Cms.Core.Web;
using Umbraco.Cms.Web.Common.Controllers;
using Umbraco.Cms.Web.Common.PublishedModels;
public class ErrorPageController : RenderController
{
public ErrorPageController(ILogger<RenderController> logger, ICompositeViewEngine compositeViewEngine,
IUmbracoContextAccessor umbracoContextAccessor)
: base(logger, compositeViewEngine, umbracoContextAccessor)
{
}
[ResponseCache(NoStore = true, Location = ResponseCacheLocation.None)]
public override IActionResult Index()
{
var errorPage = CurrentPage as ErrorPage;
Response.StatusCode = int.TryParse(errorPage.ErrorStatusCode, out var errorStatusCode) ? errorStatusCode : 500;
return CurrentTemplate(CurrentPage);
}
}
ErrorPageController.cs
By default for a 404 page Umbraco will intercept any pages not found and show you a generic page.
You can update the appsettings with the content key of your 404 page so the default 404 traffic goes to that page instead.
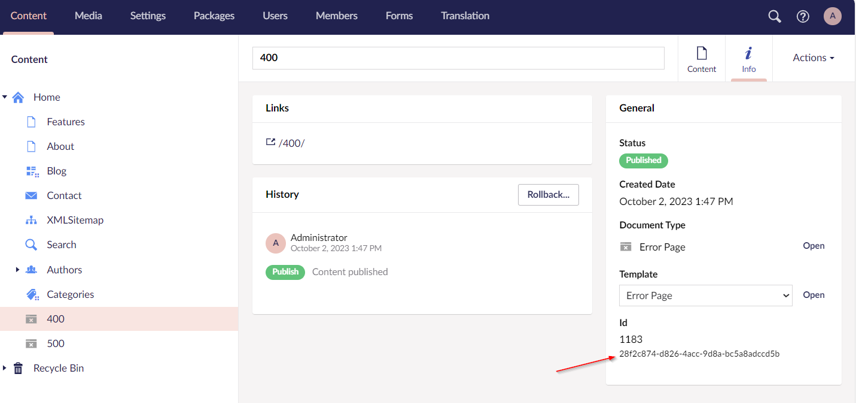
404 page content key
"Umbraco": {
"CMS": {
"Content": {
"Error404Collection": [
{
"Culture": "default",
"ContentKey": "28f2c874-d826-4acc-9d8a-bc5a8adccd5b"
}
]
},
}
}
appsettings for 404 page
Now if you have generated your models and built the code you should be able to test it all out and see that it shows your custom error pages for 500 errors and 404 errors.
You can use dev tools to inspect the headers to see if it is actually returning a 500 status code.
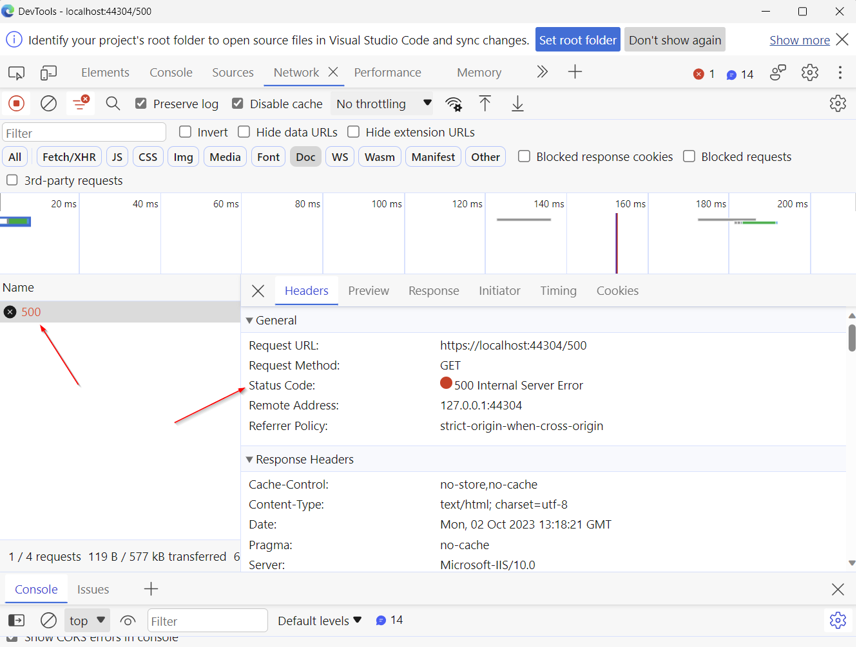
500 page with a 500 error status code
If it isn't working for you in development, it is probably because of the if statement in the Configure method of the Startup.cs file because it is set to show the raw error in development, so you might want to update it just for testing purposes.
if (env.IsDevelopment())
{
//app.UseDeveloperExceptionPage();
app.UseExceptionHandler("/error");
}
else
{
app.UseExceptionHandler("/error");
}
Use the exception handler for testing purposes in development